what is the best way to remove the vulnerability caused by buffer-overflow problems?
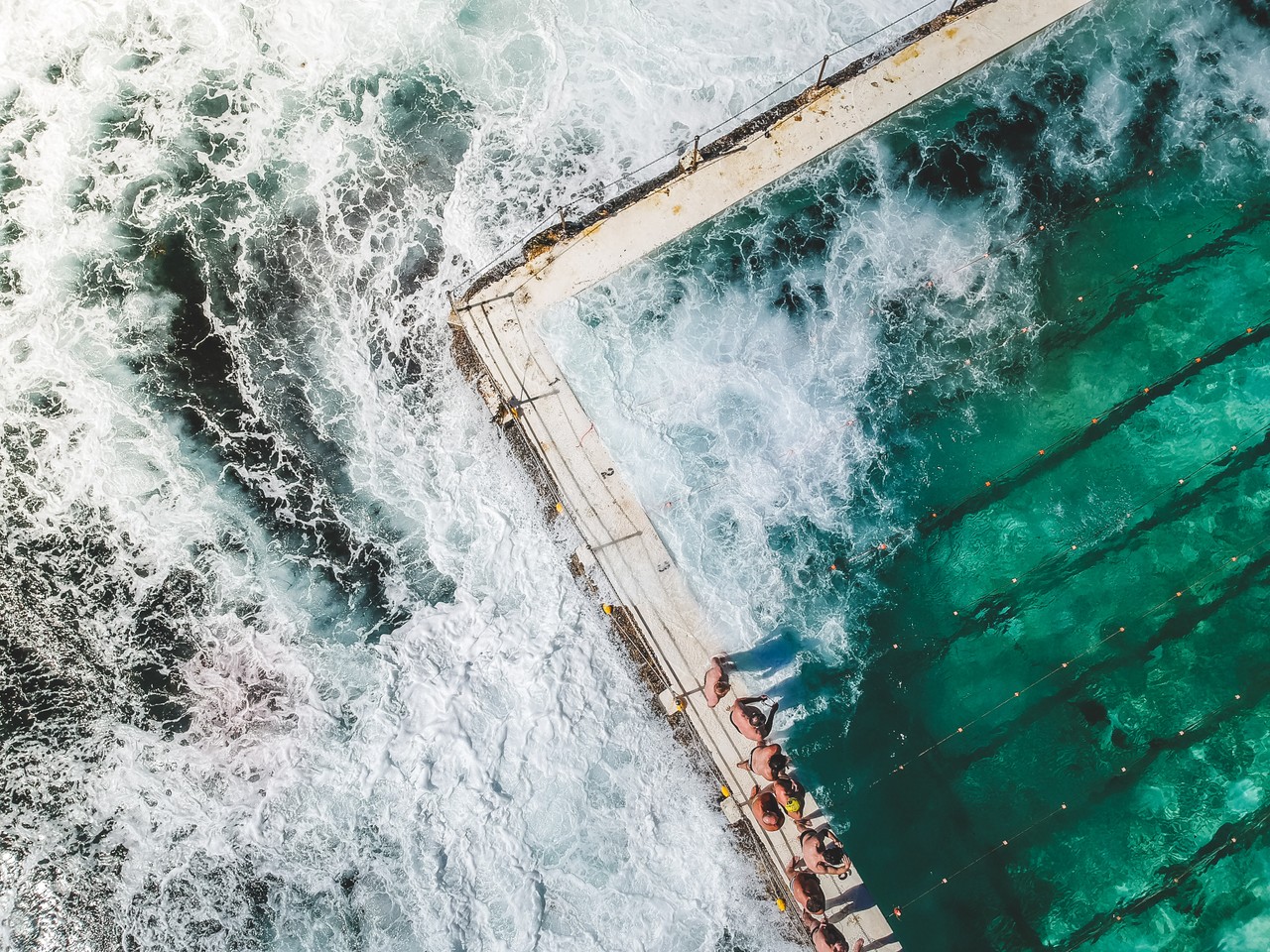
A buffer overflow occurs when the size of information written to a memory location exceeds what information technology was allocated. This can cause data corruption, program crashes, or even the execution of malicious code.
While C, C++, and Objective-C are the main languages which have buffer overflow vulnerabilities (as they deal more directly with retention than many interpreted languages), they are the foundation of much of the internet.
Even if the lawmaking is written in a 'condom' language (like Python), if it calls on any libraries written in C, C++, or Objective C, it could still be vulnerable to buffer overflows.
Memory Allocation
In order to empathise buffer overflows, it's important to sympathize a piddling about how programs allocate retention. In a C plan, y'all can allocate memory on the stack, at compile time, or on the heap, at run fourth dimension.
To declare a variable on the stack: int numberPoints = 10;
Or, on the heap: int* ptr = malloc (ten * sizeof(int));
Buffer overflows tin can occur on the stack (stack overflow) or on the heap (heap overflow).
In general, stack overflows are more than commonly exploited than heap overflows. This is because stacks contain a sequence of nested functions, each returning the address of the calling office to which the stack should return after the function has finished running. This return address can be replaced with the didactics to instead execute a piece of malicious code.
Equally heaps less commonly shop these return addresses, it's much harder to launch an exploit (though not impossible). Memory on the heap typically contains program information and is dynamically allocated equally the programme runs. This means that a heap overflow would probable accept to overwrite a part pointer – harder and less effective than a stack overflow.
As stack overflows are the more usually exploited type of buffer overflow, nosotros'll briefly dig into exactly how they work.
Stack Overflows
When an executable is run, it runs inside a process, and each process has its own stack. As the process executes the main function, it will find both new local variables (which will be pushed onto the height of the stack) and calls to other functions (which will create a new stackframe).
A diagram of a stack, for clarity:
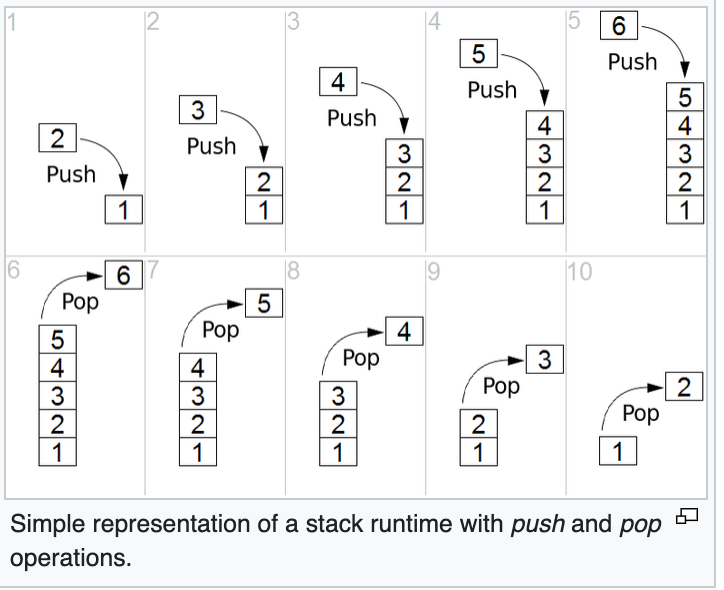
So, what'due south a stackframe?
Start, a call stack is basically the assembler code for a particular program. Information technology'southward a stack of variables and stackframes which tell the computer in what gild to execute instructions. There will be a stackframe for each function that hasn't nonetheless finished executing, with the function which is currently executing on the top of the stack.
In lodge to keep track of this, a computer keeps several pointers in memory:
- Stack Pointer: Points to the superlative of the process telephone call stack (or the last particular pushed onto the stack).
- Instruction Pointer: Points to the address of the next CPU didactics to exist executed.
- Base Arrow (BP): (likewise known as the frame pointer) Points to the base of the electric current stackframe. It stays constant as long as the program is executing the current stackframe (though the stack arrow will change).
For instance, given the post-obit program:
int master() { int j = firstFunction(5); return 0; } int firstFunction(int z) { int 10 = 1 + z; render x; }
The call stack would expect like this, right subsequently firstFunction has been called and the statement int x = 1+z
has been executed:
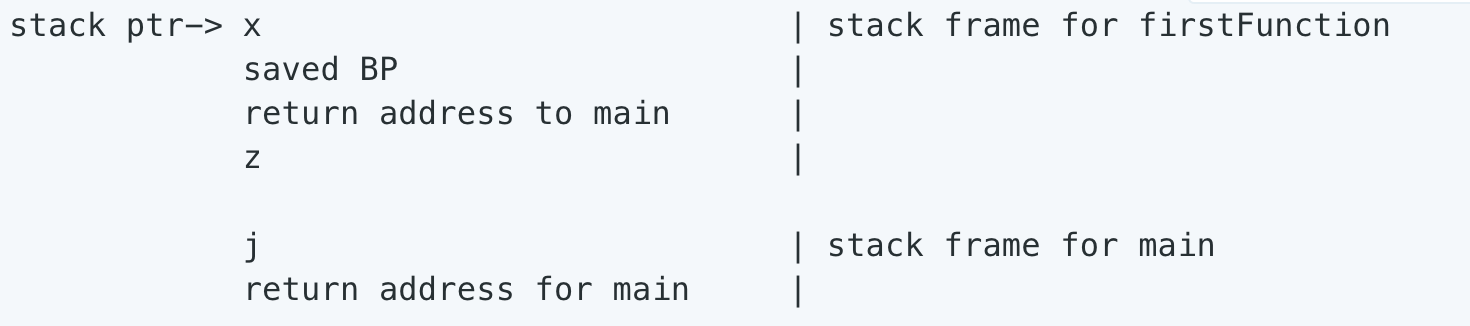
Here, primary
called firstFunction
(which is currently executing), so it's at the tiptop of the call stack. The return address is the memory accost of the office which called information technology (this is held by the instruction pointer equally the stackframe is created). Local variables which are even so in telescopic are besides on the call stack. As they are executed and get out of telescopic, they are 'popped' off the top of the stack.
Thus, the computer is able to keep rails of which instruction needs to be executed, and in which society. A stack overflow is designed to overwrite 1 of these saved return addresses with its own, malicious address.
Example Buffer Overflow Vulnerability (C):
int principal() { bufferOverflow(); } bufferOverflow() { char textLine[x]; printf("Enter your line of text: "); gets(textLine); printf("You lot entered: ", textLine); render 0; }
This simple case reads in an arbitrary amount of data (gets will read in until the end of the file or the newline character). Thinking virtually the call stack we walked through above, you can see why this is dangerous. If the user enters more than data than the amount the variable is assigned, the string the user entered will overwrite the side by side retention locations on the call stack. If it is long enough, it may even overwrite the render address of the calling function.
How the reckoner will react to this depends on how stacks are implemented and how memory is allocated in a particular organization. The response to a buffer overflow can be quite unpredictable ranging from plan faults, to crashes, to execution of malicious lawmaking.
Why Do Buffer Overflows Occur?
The reason buffer overflows became such a significant problem is that many memory manipulation functions in C and C++ don't perform any premises checking. While buffer overflows are quite well-known now, they're also very commonly exploited (for case, WannaCry exploited a buffer overflow).
Buffer overflows are near common when the code relies on external input information, is too complex for a programmer to hands empathise its behavior, or when it has dependencies outside the directly telescopic of the code.
Web servers, application servers, and spider web application environments are all susceptible to buffer overflows.
The exception is environments written in interpreted languages, though the interpreters themselves tin can be susceptible to overflows.
How to Mitigate Buffer Overflows
- Utilise an interpreted language which isn't susceptible to these issues.
- Avert using functions which don't perform buffer checks (for instance, in C, instead of gets() utilise fgets()).
- Use compilers which can help place unsafe functions or errors.
- Use Canaries, a 'guard value' which can assistance preclude buffer overflows. They're inserted before a return address in the stack and are checked before the render address is accessed. If the program detects a alter to the canary value, it will arrest the process, preventing the assaulter from succeeding. The canary value is either random (and then, very difficult for an attacker to gauge) or a string of characters which, for technical reasons, is impossible to overwrite.
- Re-arrangement of local variables so scalar variables (individual fixed-size data objects) are above array variables (containing multiple values). This means that if the assortment variables do overflow, they won't impact the scalar variables. This technique, when combined with canary values, can help prevent buffer overflow attacks from succeeding.
- Make a stack non-executable by setting the NX (No-eXecute) flake, preventing the assailant from inserting shellcode directly into the stack and executing it there. This isn't a perfect solution, as even not executable stacks tin be victims of buffer overflow attacks such as the return-to-libc attack. This assail occurs when the return accost of a stackframe is replaced with the accost of a library already in the procedure' address infinite. Additionally, non all CPUs allow for the NX bit to be set.
- ASLR (address infinite layout randomization), can serve as a general defense (as well as a specific defense against render-to-libc attacks). It means that someday a library file or other office is chosen by a running process, its address is shifted past a random number. Information technology makes information technology about impossible to associate a fixed process memory accost with functions, meaning that information technology tin exist difficult, if not incommunicable, for an attacker to know from where to call specific functions. ASLR is on by default in many versions of Linux, Bone Ten, and Android (which tin can be toggled off in the command line).
Annotation on Stack Underflow:
It's besides possible to accept a buffer underflow vulnerability, when two parts of the same program care for the same cake of memory differently. For case, if you lot classify an array of size 10, but fill information technology with an array of size ten < 10, and later you endeavor to retrieve all Ten bytes, yous're probable to go garbage data for X - 10 bytes.
Substantially y'all may accept pulled data which is left over from how that retention was previously used. The best case is that it'due south garbage that doesn't mean annihilation, while the worst case is that it is sensitive data that an aggressor might exist able to misuse.
Sources/Further Reading:
- Computer and Network Security Lectures, Avinash Kak
- OWASP Buffer Overflows
- Stack vs Heap
Learn to lawmaking for gratis. freeCodeCamp's open source curriculum has helped more than twoscore,000 people get jobs equally developers. Get started
lockhartchemb1995.blogspot.com
Source: https://www.freecodecamp.org/news/buffer-overflow-attacks/
0 Response to "what is the best way to remove the vulnerability caused by buffer-overflow problems?"
ارسال یک نظر